CS 696: Advanced OO Programming and Design
Spring Semester, 1997
Doc 1, Introduction
[To Lecture Notes Index]
San Diego State University -- This page last updated Jan 27, 1997

Contents of Doc 1, Introduction
- References
- Introduction
- Meyer's Criteria for Evaluating for Modularity
- Principles for Software Development
- What is Object-Oriented Programming
- Language Level Definition of OOP
- Conceptual Level Definition of OOP
- Stages of Object-Oriented Expertise2
- Some Heuristics
Object-Oriented Software Construction, Bertrand Meyer, Prentice Hall,
1988
Object-Oriented Design Heuristics, Arthur Riel, Addison-Wesley, 1996
Decomposability
Decompose problem into smaller subproblems
that can be solved separately
Example: Top-Down Design
Counter-example: Initialization Module
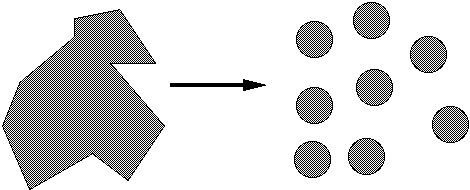
Meyer's Criteria for Evaluating for Modularity
Composability
Freely combine modules to produce new systems
Examples: Math libraries
Unix command & pipes
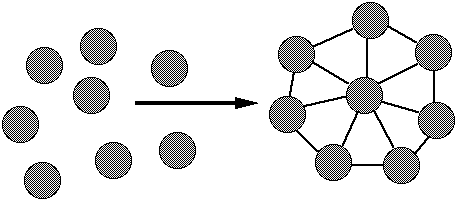
Meyer's Criteria for Evaluating for Modularity
Understandability
Individual modules understandable by human reader
Counter-example: Sequential Dependencies
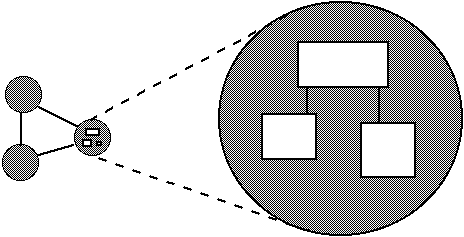
Meyer's Criteria for Evaluating for Modularity
Continuity
Small change in specification results in:
Changes in only a few modules
Does not affect the architecture
Example: Symbolic Constants
const MaxSize = 100
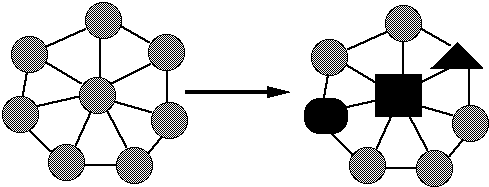
Meyer's Criteria for Evaluating for Modularity
Protection
Effects of an abnormal run-time condition is confined to a few modules
Example: Validating input at source
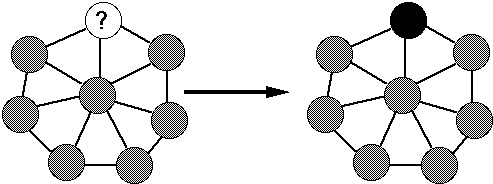
KISS Keep it simple, stupid
Supports:
-
- Understandablity
- Composability
Decomposability
Small is Beautiful
Upper bound for average size of an operation[1]
Language | Lines of Code |
Smalltalk | 8 |
C++ | 24 |
Supports:
- Decomposability
- Composability
- Understandability
Applications of Principles
First program:
class HelloWorldExample
{
public static void main( String args[] )
{
System.out.println( "Hello World" );
}
}
Grow programs
- Start with working program
-
- Add small pieces of code and debug
-
-
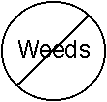
Language Level Definition
Conceptual Level Definition
Object
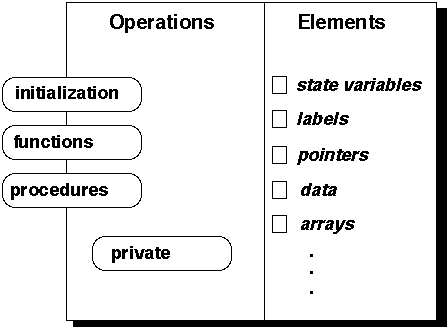
Language Level Definition of OOP
Class
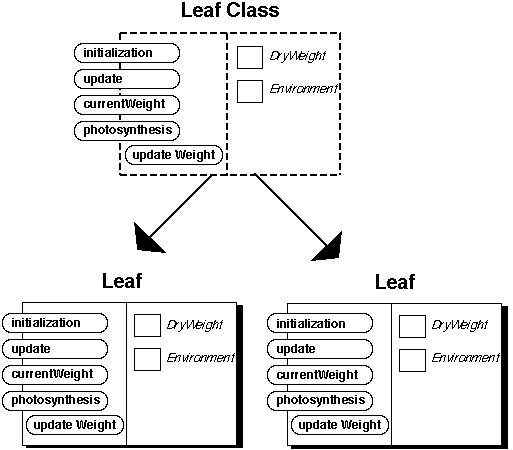
Language Level Definition of OOP
Inheritance
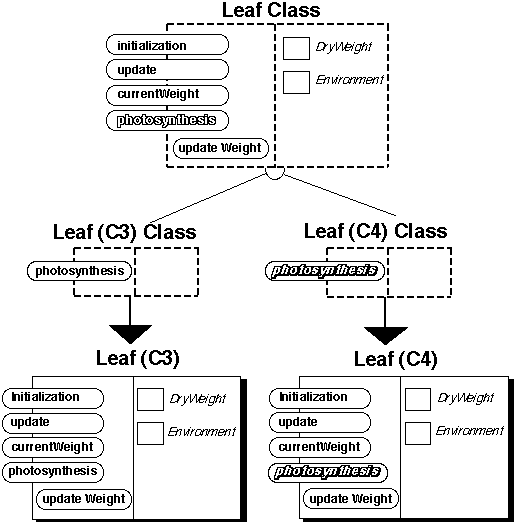
Abstraction
"Extracting the essential details about an item or group of items, while
ignoring the unessential details."
Edward Berard
"The process of identifying common patterns that have systematic variations; an
abstraction represents the common pattern and provides a means for specifying
which variation to use."
Richard Gabriel
Example
Pattern: Priority queue
Essential Details: length
items in queue
operations to add/remove/find item
Variation: link list vs. array implementation
stack, queue
Conceptual Level Definition of OOP
Encapsulation
Enclosing all parts of an abstraction within a container
Example
Conceptual Level Definition of OOP
Information Hiding
Hiding parts of the abstraction
Example
Conceptual Level Definition of OOP
Hierarchy
Abstractions arranged in order of rank or level
Class Hierarchy
Conceptual Level Definition of OOP
Hierarchy
Object Hierarchy
Novice
Duration: 3 to 6 months
"Novices spend their time trying to get their function-oriented heads turned
around, so they can see the application object classes. They have a hard time
finding the classes and write a lot of function-oriented code."
Apprentice
Duration: 3 to 6 months
"Apprentices begin to understand what object-orientation really means. They
still prefer to work with others, who help foster an environment of discovery.
Apprentices begin turning out good designs, although not consistently. Gurus
and journeymen still need to keep an eye on apprentices."
Stages of Object-Oriented Expertise
Journeyman
Duration: varies greatly, a least a year
"Journeyman have internalized the OO paradigm and work independently. They can
provide key inputs during the design reviews. They still have mental
roadblocks that may require a guru to break through."
Guru
Duration: lifetime
"For gurus, OO development comes naturally. A guru automatically sees things
in an OO perspective, moving quickly through the phases of development during
rapid iterations. A guru can move the project over mental hurdles, causing
others to see something clearly that eluded them before."
"There are very few gurus in the world today. The rest of us will have to rely
on proven processes and methodologies."
"I've yet to see someone successfully make it past the novice stage and even
consider thinking about software in other than OO terms."
A class should capture one and only one abstraction
- Class = abstraction
Keep related data and behavior in one place
- An abstraction is both data and behavior (methods)
All data should be hidden within its class
-
- Information hiding
Heuristics Continued
Beware of classes that have many accessor methods defined in their public
interface. Having many implies that related data and behavior is not being
kept in one place.
class test
{
private int thisData;
private int thatData;
private float moreData;
public void setThisData( int data ) { thisData = data; }
public void setThatData( int data ) { thatData= data; }
public void setMoreData( int data ) { moreData= data; }
public void getThisData( ) { return thisData; }
public void getThatData( ) { return thatData; }
public void getMoreData( ) { return moreData; }
public String toString() { // code deleted }
}
No work is being done in this class.
Other classes are getting the data in class test and performing some operation
on it.
Why is this class not doing the work on the data!
Who is doing the work?
The God Class Problem
Distribute system intelligence horizontally as uniformly as possible, that is,
the top-level classes in a design should share the work uniformly.
Do not create god classes/objects in your system. Be very suspicious of a class
whose name contains Driver, Manager, System, or
Subsystem
Beware of classes that have too much noncommunicating behavior, that is,
methods that operate on a subset of the data members of a class. God classes
often exhibit much noncommunicating behavior.
Divide noncommunicating behavior into separate classes
The God Class Problem - Data Form
Legacy Non-OO System
Poor Migration to OO System
Proliferation of Classes Problem
Be sure that the abstractions that you model are classes and not simply roles
object play
Do we use a Father class, Mother Class, and a Child class to model a family?
Or do we use a Person class, with Person objects acting as Father, Mother and
Child?
Do not turn an operation into a class.
Be suspicious of any class whose name is a verb or is derived from a verb,
especially those that have only one piece of meaningful behavior ( i.e. do not
count sets, gets, prints).